マルチAZのRedshiftクラスターをCDKで実装する
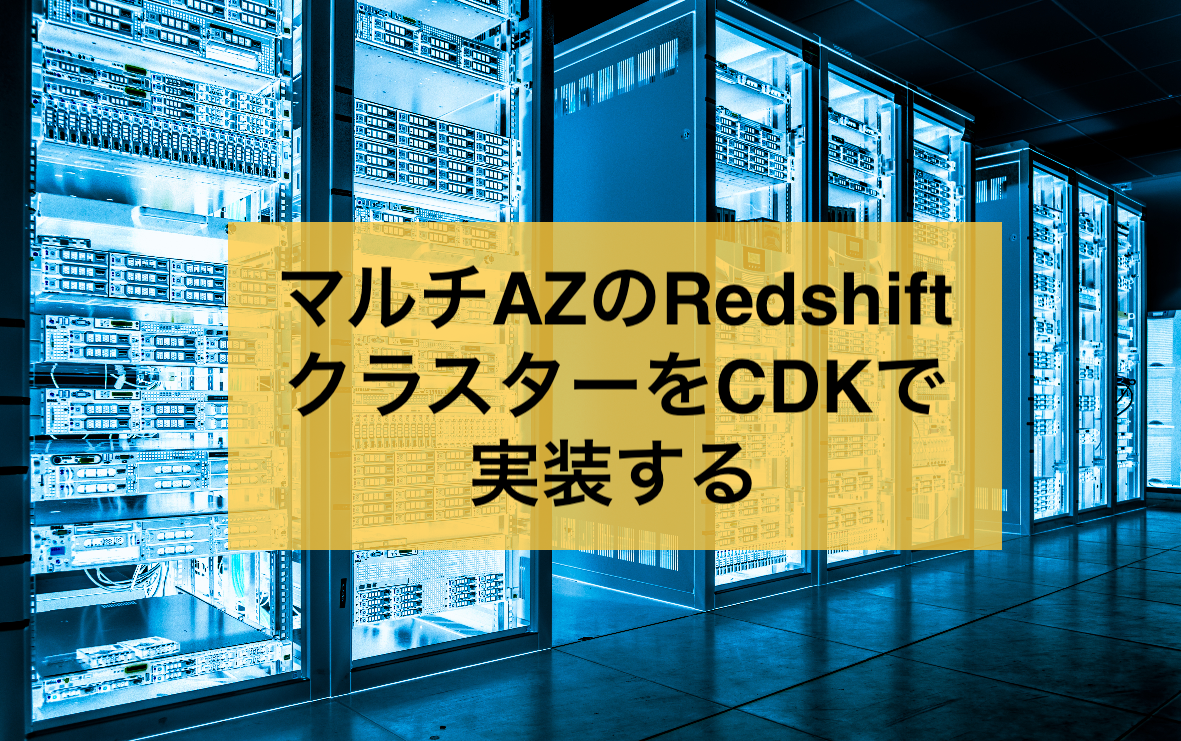
Amazon RedshiftのマルチAZは、約1年前の2023年11月1日に一般提供が開始されました。
比較的新しい機能ということもあり、いくつか制約とCDKで実装する際のハマリポイントがあったため、備忘録として残します。
マルチAZの制約
CDKで新規にマルチAZのRedshiftクラスターを構築する際に留意すべきポイントは以下です。
- RA3インスタンスタイプのみサポート
- 暗号化必須
- 二つ以上のノード必須
- 3AZのサブネット必須
RDSサブネットグループは2AZ以上ですが、Redshiftのサブネットグループの場合、3AZのサブネットを指定してあげる必要があるので留意が必要です。
具体的な制約は以下公式ドキュメントをご参照ください。
マルチAZ時の最低コスト
上記制約から、マルチAZで構築する場合、RA3インスタンスタイプで2ノード*2AZ=4ノードが必要となります。
直近の2024年10月1日にRA3系の最小インスタンスであるRA3.largeがリリースされましたが、最小インスタンスを使用する場合でも、コストに留意する必要があります。
Amazon Redshift が RA3.large インスタンスをリリース
https://aws.amazon.com/jp/about-aws/whats-new/2024/10/amazon-redshift-ra3-large/
下記の通り、見積もりが$1,865.88/月となります。
CDKでマルチAZのRedshiftクラスターを構築
制約を加味すると以下のような実装になります。
import * as cdk from "aws-cdk-lib";
import { Construct } from "constructs";
import * as redshift from "aws-cdk-lib/aws-redshift";
import * as ec2 from "aws-cdk-lib/aws-ec2";
export class RedshiftMultiAzStack extends cdk.Stack {
constructor(scope: Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
// Redshift用のVPCを作成
const vpc = new ec2.Vpc(this, "RedshiftVPC", {
maxAzs: 3, // 3AZのサブネット必須
natGateways: 0,
subnetConfiguration: [
{
cidrMask: 24,
name: "private-isolated",
subnetType: ec2.SubnetType.PRIVATE_ISOLATED,
},
],
});
// Redshift用のセキュリティグループを作成
const securityGroup = new ec2.SecurityGroup(
this,
"RedshiftSecurityGroup",
{
vpc: vpc,
description: "Security group for Redshift cluster",
allowAllOutbound: true,
}
);
// ポート5439(Redshiftのデフォルトポート)へのインバウンド許可
securityGroup.addIngressRule(
ec2.Peer.anyIpv4(), // 本番環境では絞ることを推奨
ec2.Port.tcp(5439),
"Allow Redshift access"
);
// Redshift用のサブネットグループを作成
const subnetGroup = new redshift.CfnClusterSubnetGroup(
this,
"RedshiftSubnetGroup",
{
description: "Subnet group for Redshift cluster",
subnetIds: vpc.selectSubnets({
subnetType: ec2.SubnetType.PRIVATE_ISOLATED,
}).subnetIds,
}
);
// Redshiftクラスターを作成
new redshift.CfnCluster(this, "RedshiftCluster", {
multiAz: true,
encrypted: true, // 暗号化必須
clusterType: "multi-node",
nodeType: "ra3.large", // RA3インスタンスタイプのみサポート
numberOfNodes: 2, // 二つ以上のノード必須
masterUsername: "admin",
masterUserPassword: "AdminPassword123", // 実運用ではSecrets Managerの使用を推奨
dbName: "multiazdb",
clusterSubnetGroupName: subnetGroup.ref, // 3AZのサブネット必須
vpcSecurityGroupIds: [securityGroup.securityGroupId],
publiclyAccessible: false,
});
}
}
CDKで3AZを実装する際のハマりポイント
下記AWS CDK Referenceに記載されているように、3AZ以上を作成する場合は、accountとregionを指定してあげる必要があります。
https://docs.aws.amazon.com/cdk/api/v1/docs/@aws-cdk_aws-ec2.Vpc.html#maxazs
Be aware that environment-agnostic stacks will be created with access to only 2 AZs, so to use more than 2 AZs, be sure to specify the account and region on your stack.
そのため、以下のようにenvを追加する必要があります。
#!/usr/bin/env node
import "source-map-support/register";
import * as cdk from "aws-cdk-lib";
import { RedshiftMultiAzStack } from "../lib/redshift-multi-az-stack";
const app = new cdk.App();
const env = {
account: process.env.CDK_DEFAULT_ACCOUNT,
region: process.env.CDK_DEFAULT_REGION,
};
new RedshiftMultiAzStack(app, "RedshiftMultiAzStack", { env });
まとめ
DC2インスタンスタイプからマルチAZに移行する場合はコストが跳ね上がり、気軽にマルチAZ化できないので留意しましょう。